As we delve into the realm of advanced Bash scripting, we’re embarking on a journey that will elevate our command-line prowess to new heights. Whether you’re a seasoned system administrator or an aspiring DevOps engineer, mastering these techniques will empower you to create more efficient, robust, and sophisticated scripts. In this comprehensive guide, we’ll explore the intricacies of Bash scripting, unraveling complex concepts and providing practical examples to enhance your scripting skills.
Throughout this tutorial, we’ll cover a wide array of topics, from optimizing script performance to implementing error handling mechanisms. We’ll dive deep into advanced data structures, explore powerful text processing techniques, and uncover the secrets of automating complex system administration tasks. By the end of this guide, you’ll have a solid foundation in advanced Bash scripting, enabling you to tackle even the most challenging automation projects with confidence.
If you need a basic brush up on the skills then I will recommend the article here at: https://www.freecodecamp.org/news/bash-scripting-tutorial-linux-shell-script-and-command-line-for-beginners/
So, let’s roll up our sleeves and dive into the fascinating world of advanced Bash scripting. Get ready to unlock the full potential of your command-line skills and take your scripting expertise to the next level!
Advanced Linux Shell Scripting for Server Management
Linux shell scripting is a powerful tool for automating system administration and server management tasks. Advanced shell scripting involves complex logic, error handling, performance optimizations, and integration with other tools like Python, awk, and sed. In this article, we will explore real-life examples of advanced shell scripting for server management.
So even a simple script like this:
#!/bin/bash
LOGFILE="/var/log/server_health.log"
echo "Server Health Check - $(date)" >> $LOGFILE
# Check CPU Usage
CPU_USAGE=$(top -bn1 | grep "Cpu(s)" | awk '{print $2 + $4}')
echo "CPU Usage: $CPU_USAGE%" >> $LOGFILE
# Check Memory Usage
MEMORY=$(free -m | awk 'NR==2{printf "%.2f%%", $3*100/$2 }')
echo "Memory Usage: $MEMORY" >> $LOGFILE
# Check Disk Space
DISK_USAGE=$(df -h / | awk 'NR==2 {print $5}')
echo "Disk Usage: $DISK_USAGE" >> $LOGFILE
# Check Running Services
SERVICES=(nginx mysql sshd)
for SERVICE in "${SERVICES[@]}"; do
systemctl is-active --quiet $SERVICE
if [ $? -ne 0 ]; then
echo "$SERVICE is not running!" >> $LOGFILE
fi
done
echo "Health check completed. Log file: $LOGFILE"
which provides you a complete health check of your website will save you lot of work if you have to run it through a larger span of server cluster. So lets try to learn this better.
Harnessing the Power of Arrays in Bash
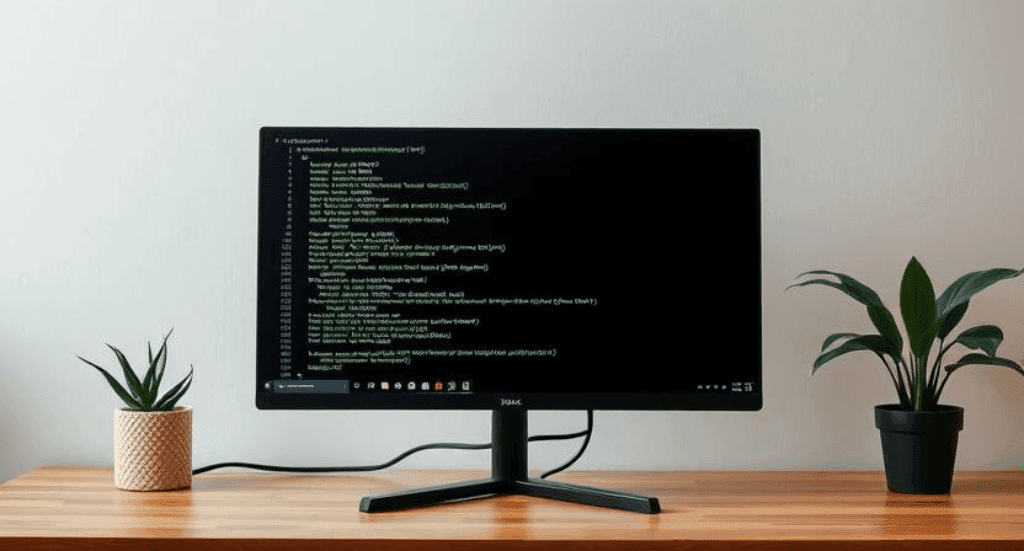
Arrays in Bash provide a powerful mechanism for managing collections of data within your scripts. By leveraging arrays effectively, you can streamline your code and handle complex data structures with ease. Let’s explore the various aspects of working with arrays in Bash and discover how they can enhance your scripting capabilities.
Declaring and Initializing Arrays
In Bash, arrays offer flexibility in how they can be declared and initialized. You can create an array by simply assigning values to it using parentheses. For instance:
fruits=("apple" "banana" "cherry" "date")
This creates an array named fruits
with four elements. Alternatively, you can declare an array explicitly using the declare
command:
declare -a numbers
This creates an empty array named numbers
. You can then add elements to it individually:
numbers[0]=10
numbers[1]=20
numbers[2]=30
Accessing and Manipulating Array Elements
Retrieving values from an array is straightforward. You can access individual elements using their index:
echo ${fruits[1]} # Outputs: banana
To access all elements of an array, you can use the @
or *
notation:
echo ${fruits[@]} # Outputs: apple banana cherry date
Bash also provides various parameter expansions that allow you to manipulate arrays in powerful ways. For example, you can slice an array:
echo ${fruits[@]:1:2} # Outputs: banana cherry
This retrieves two elements starting from index 1.
Adding and Removing Array Elements
You can append elements to an array using the +=
operator:
bashfruits+=("elderberry")
To remove an element, you can use the unset
command:
bashunset fruits[2]
This removes the element at index 2 (cherry in our example).
Iterating Over Arrays
Looping through array elements is a common operation in Bash scripting. You can use a for
loop to iterate over all elements:
for fruit in "${fruits[@]}"; do
echo "I like $fruit"
done
This will print a message for each fruit in the array.
Associative Arrays
Bash 4.0 and later versions support associative arrays, which allow you to use strings as keys instead of numeric indices. Here’s how you can declare and use an associative array:
declare -A colors
colors[sky]="blue"
colors[grass]="green"
colors[sun]="yellow"
echo "The sky is ${colors[sky]}"
Associative arrays provide a convenient way to create key-value pairs in your scripts.
For example you can easily use the array operations on a simple script like:
#!/bin/bash
SERVERS=("server1.example.com" "server2.example.com" "server3.example.com")
LOG_DIR="/var/log/remote_logs"
mkdir -p $LOG_DIR
for SERVER in "${SERVERS[@]}"; do
echo "Fetching logs from $SERVER"
scp user@$SERVER:/var/log/syslog $LOG_DIR/syslog_$SERVER.log
scp user@$SERVER:/var/log/auth.log $LOG_DIR/auth_$SERVER.log
done
# Analyze logs
for LOG_FILE in $LOG_DIR/*.log; do
echo "Analyzing $LOG_FILE"
grep -i "error" $LOG_FILE | tee -a $LOG_DIR/summary.log
done
echo "Log collection and analysis completed."
By mastering these array techniques, you’ll be able to handle complex data structures more efficiently in your Bash scripts. Arrays can significantly simplify your code when dealing with collections of data, making your scripts more readable and maintainable.
We will be digging deep in this series on more advanced techniques of regex word processing and more deeper understanding on how you can become a better devops engineer.
The part 2 of this series is here
https://pixelhowl.com/advanced-shell-scripting-part-2-automation-server-management/
Stay Tuned!
[…] Continuing with our take on advanced shell scripting using bash – we are diving in deeper. You can find the first tutorial here at: Advanced Shell Scripting – part 1 […]
[…] Part 1: https://pixelhowl.com/advanced-shell-scripting-master-class/ […]
[…] Chatter 1: https://pixelhowl.com/advanced-shell-scripting-master-class. […]