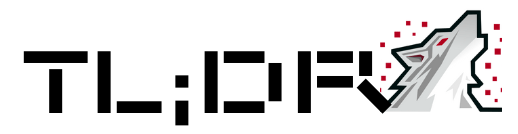
Getting our deployment time under 15 minutes was critical for our team. GitHub Actions CI/CD made this possible, though the journey wasn’t straightforward at first.
The old days of CI/CD setup were quite challenging. We remember spending weeks configuring servers and writing deployment scripts from scratch. GitHub Actions changed everything for us. The marketplace now offers over 13,000 ready-to-use workflows, making our build and deploy process much simpler right within our code repository.
The switch to GitHub Actions proved excellent for our development workflow. Our requirements were specific – we needed a platform working with multiple technology stacks while keeping our developers focused on feature development rather than deployment tasks. GitHub Actions matched these requirements perfectly.
The setup process wasn’t complex once we understood the basics. Whether you’re just starting with continuous integration or looking to improve your current setup, this guide covers everything we learned during our implementation. We’ll share the exact steps that worked for us, helping you avoid the common pitfalls we encountered along the way.
Understanding GitHub CI/CD Pipeline
Our team needed a reliable way to automate code testing and deployment. GitHub CI/CD pipeline turned out to be the perfect solution, handling everything from testing new code to pushing updates to users. The automation runs right inside our GitHub repository (quite convenient for our developers).
The process works seamlessly – developers push their code changes, and the CI part jumps into action testing everything automatically. Once tests pass, CD takes over and deploys the changes. The speed improvement was remarkable, especially compared to our old manual deployment process.
Key Parts We Use
Setting up the pipeline meant understanding several connected pieces:
- Workflow Files: These YAML files (took us some time to get used to the syntax) tell GitHub exactly what to do with our code.
- Jobs and Steps: Think of jobs as separate tasks running on different machines. Each job has specific steps – from running our test suite to deploying the application.
- Triggers: Our workflows start automatically when specific things happen, like new pull requests or code commits.
- Runner Machines: These are the actual servers running our workflows. GitHub provides Linux, Windows, and macOS options (we mainly stick to Linux for our needs).
Why GitHub Actions Works for Us
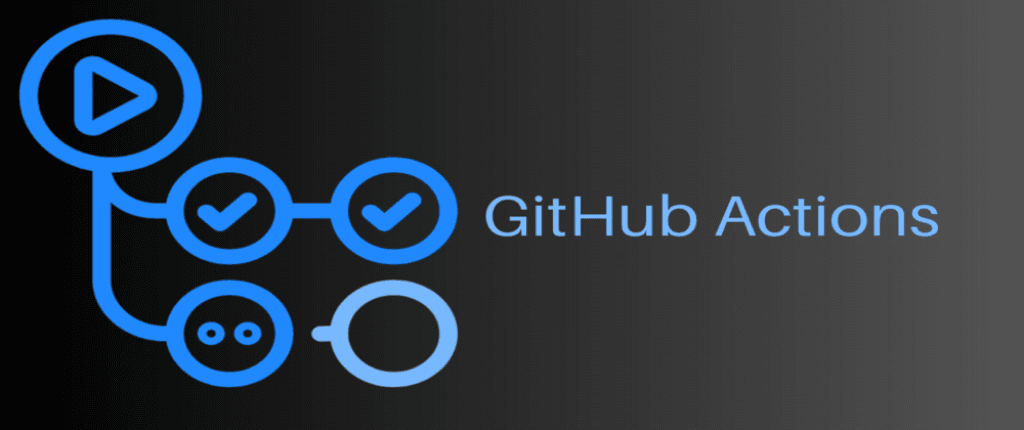
The benefits we discovered while using GitHub Actions were quite impressive:
Built-in Integration: Everything happens right where our code lives. No more juggling between different tools. This integration was a huge time-saver for our team.
Works with Everything: Our stack requirements were specific, but GitHub Actions handled them all perfectly. The webhook support just added to its flexibility.
Ready-made Solutions: The marketplace (with over 11,000 pre-built workflows) saved us from writing everything from scratch. The community contributions were really helpful.
Budget Friendly: Being a cost-conscious team, we appreciated the free tier for public repositories and reasonable limits for private ones. The option to host our own runners was a nice bonus.
Easy Setup: The learning curve wasn’t steep at all. Our developers got comfortable with it quickly, even those who hadn’t worked with CI/CD before.
The early error detection really improved our code quality. The continuous testing meant fewer issues making it to production (a relief for our support team).
We customized our workflows to match exactly what we needed – from code style checks to security scans and deployment rules. The flexibility reminded us of our initial hosting requirements, where customization options were crucial for our success.
Setting Up GitHub Environment

Getting our GitHub environment properly configured was quite challenging at first. Security requirements were specific, and we had to be extra careful with access controls and permissions.
>Tools We Needed
1. Admin Access to GitHub Repository
- To configure CI/CD pipelines, you need admin-level permissions on the GitHub repository.
- This allows you to:
- Enable GitHub Actions.
- Configure secrets (like API keys or tokens).
- Modify repository settings and workflows.
- If you’re not the admin, you may need to request the required permissions from your organization.
2. Personal Access Token (PAT) for Secure Access
- A Personal Access Token (PAT) is required to authenticate external systems with GitHub securely.
- This is especially useful when:
- Automating deployments to cloud platforms.
- Accessing private repositories from CI/CD pipelines.
- Running workflows that need elevated permissions beyond the default GitHub Actions token.
- You can create a PAT by:
- Going to GitHub > Settings > Developer Settings > Personal Access Tokens.
- Selecting the required scopes (e.g.,
repo
for repository access,workflow
for GitHub Actions). - Storing the token securely in GitHub Secrets.
3. GitHub Actions (Enabled in Repository Settings)
- GitHub Actions must be enabled to allow automation of builds, tests, and deployments.
- To enable:
- Navigate to Repository Settings > Actions.
- Ensure that “Allow all actions” is selected (or define allowed workflows).
- This lets you define workflows inside
.github/workflows/
using YAML files.
4. Access to Marketplace Workflows
- GitHub has a Marketplace where you can use pre-built workflows, such as:
- Linting (e.g., ESLint, Prettier for JavaScript projects).
- Testing (e.g., Jest, PHPUnit, Pytest).
- Deployment (e.g., AWS, Azure, DigitalOcean).
- You can add workflows from the Marketplace by:
- Visiting https://github.com/marketplace.
- Searching for an action (e.g., “Deploy to AWS”).
- Clicking “Use latest version” to add it to your repository’s workflow file.
5. Development Setup Matching Production Environment
- Your local development environment should match your production setup to ensure consistent builds and deployments.
- This includes:
- Same OS and dependencies: If your production runs on Ubuntu 22.04, use the same version locally.
- Matching runtime versions: Node.js, Python, PHP, or other languages should be the same.
- Similar database configurations: If using MySQL, PostgreSQL, or MongoDB, match settings with production.
- Docker (if used in production): If production runs containers, ensure local dev uses the same Docker images.
This setup ensures a smooth and reliable CI/CD workflow by keeping everything in sync between development, staging, and production.
>Repository Setup Steps
1. Actions Setup
GitHub Actions is typically enabled by default for repositories, but it’s always good to verify:
- Check if Actions is Enabled
- Navigate to your repository on GitHub.
- Go to Settings > Actions.
- Ensure that the Actions permissions are set to allow workflow runs:
- Allow all actions and reusable workflows (default).
- Alternatively, Restrict actions to specific workflows if security is a concern.
- Verify Default Runner Access (Optional)
- If you’re using GitHub-hosted runners, you don’t need additional setup.
- If using self-hosted runners, you’ll need to register a runner via Settings > Actions > Runners.
2. Token Configuration
Since GitHub Actions needs secure access to external services (e.g., cloud platforms, private repos), a Personal Access Token (PAT) is required. As discussed earlier here in step 1.3 you can setup the PAT.
Storing the PAT Securely
- Go to Repository Settings
- Open your repository on GitHub.
- Navigate to Settings > Secrets and Variables > Actions.
- Create a New Secret
- Click New Repository Secret.
- Name it something meaningful (e.g.,
GH_PAT
). - Paste the copied token into the value field.
- Click Save.
- Add Token to Repository Settings (Critical Step)
- Some workflows need access beyond GitHub Actions (e.g., pushing to another repo).
- Go to Settings > Deploy Keys.
- Add your public SSH key if required for additional authentication.
3. Workflow Structure
GitHub Actions workflows are stored in a specific directory inside the repository.
Creating the Workflow Directory
Clone your repository locally:
git clone https://github.com/pixelhowls/action-lab.git cd gitlabs-repo
replace our git with your repo details
Create the .github/workflows
directory:
mkdir -p .github/workflows
Add your first workflow file (e.g., ci.yml
):
name: CI Pipeline on: push: branches: - main jobs: build: runs-on: ubuntu-latest steps: - name: Checkout repository uses: actions/checkout@v4
Commit and push the changes using:
git add .github/workflows/ci.yml git commit -m "Added GitHub Actions workflow" git push origin main
4. Environment Setup
Having separate staging and production environments ensures stability before deploying live.
Staging Setup
Create a staging branch (if not already set up):
git checkout -b staging git push origin staging
Adjust GitHub Secrets & Variables for staging:
- Go to Settings > Environments.
- Click New environment → Name it
staging
. - Add secrets specific to staging (e.g.,
STAGING_API_KEY
).
Production Setup
- Create a production environment in GitHub:
- Go to Settings > Environments.
- Click New environment → Name it
production
. - Add secrets specific to production (e.g.,
PROD_DB_URL
).
- Ensure staging mirrors production:
- Use the same OS and runtime (e.g., Ubuntu 22.04, Node.js 18, etc.).
- Match network configurations (e.g., firewall rules, load balancing).
- Keep dependencies identical.
>Making It Secure
Since CI/CD pipelines interact with repositories, secrets, and deployment environments, security is a critical factor. The following measures ensure access control, secrets management, workflow protection, and runner security.
Access Management (Least Privilege Model)
Proper access control prevents unauthorized changes and limits the potential attack surface.
Kept GITHUB_TOKEN
Permissions Minimal
GITHUB_TOKEN
is an automatically generated token that GitHub Actions provides to authenticate workflows.- By default, it has write access to the repository, which can be risky.
- To restrict its permissions:
- Go to Repository > Settings > Actions > General
- Under Workflow permissions, select:
- Read repository contents (instead of full write access).
- Only manually escalate permissions where absolutely necessary (e.g., deployments).
- Use fine-grained PATs for specific tasks instead of giving broad access.
Set Repository-Specific Rules
- Implemented branch protection rules to restrict direct pushes to critical branches.
- Restricted who can trigger workflows (e.g., only allow maintainers).
- Used CODEOWNERS to enforce required reviews on PRs.
Regular Permission Reviews
- Scheduled monthly access audits to check if:
- Old users still have access.
- Unused secrets should be revoked.
- Any workflows have unnecessary permissions.
Handling Secrets (Zero Trust Approach)
Secrets (API keys, database credentials, cloud tokens) should never be hardcoded in workflows or stored in plain text.
Used GitHub Secrets for Sensitive Data
- Instead of embedding credentials in
.yml
files, secrets were stored securely:- Go to Repository > Settings > Secrets and Variables > Actions.
- Click New Repository Secret.
- Add keys (e.g.,
AWS_ACCESS_KEY
,PROD_DB_URL
). - Reference them in workflows like:
env: AWS_SECRET_ACCESS_KEY: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
Encrypted Everything Important
- Used Secret Scanning (enabled by default in GitHub Advanced Security) to detect leaks.
- Never stored secrets in logs or output—used environment variables instead.
- Used GPG encryption for local storage of sensitive files before sharing.
Avoided Plain-Text Secrets Completely
- Secrets were never stored in the repository (even in old commits).
- Used tools like SOPS (Mozilla’s Secrets Ops) for managing encrypted secrets in Git.
Workflow Protection (Securing GitHub Actions)
Since workflows execute code automatically, they must be secured against unauthorized modifications and exploits.
Pinned Action Versions (Learned This the Hard Way)
- Instead of using dynamic versions:
uses: actions/checkout@v4
- Used SHA-pinned versions to prevent supply chain attacks:
uses: actions/checkout@v4 with: ref: sha256:abcd1234... # Pin exact version
Limited Permissions to Essentials
- Used the
permissions
key in workflows to restrict default permissions:
jobs: deploy: runs-on: ubuntu-latest permissions: contents: read # Only read access id-token: write # Needed for OIDC authentication
Added Branch Protection
- Enforced protected branches to prevent unauthorized workflow modifications:
- Go to Repository > Settings > Branches.
- Select Add branch protection rule.
- Enable:
- ✅ Require status checks before merging
- ✅ Require signed commits
- ✅ Restrict who can push to this branch
Environment Rules (Deployment Security)
GitHub environments allow you to define rules for production, staging, and other deployment stages.
Required Reviews for Deployments
- Used GitHub Environments to enforce manual approvals before production deployments:
- Go to Repository > Settings > Environments.
- Click New Environment (e.g.,
production
). - Enable Required Reviewers.
🔹 Added Wait Timers Where Needed
- Prevented accidental rapid deployments by adding wait times (e.g., 30 minutes between deployments).
- Used
sleep
in workflows:
- name: Wait before deployment run: sleep 1800 # Wait for 30 minutes
Set Strict Branch Policies
- Prevented merges without passing tests.
- Enabled force-push restrictions on
main
andproduction
branches.
Runner Protection (Execution Security)
Workflows run on GitHub-hosted or self-hosted runners. Protecting them ensures secure execution.
Stuck with GitHub-Hosted Runners
- Used GitHub-hosted runners instead of self-hosted ones, since GitHub:
- Provides fresh virtual machines for every job.
- Ensures no state persistence across jobs (reducing attack surface).
Fresh Environments for Each Job
- Ensured clean builds by avoiding cache poisoning:
runs-on: ubuntu-latest with: clean: true # Enforce fresh environment
Regular Security Updates
- Ensured dependencies were always up to date:
- Used
npm audit fix
,pip install --upgrade
, etc. - Regularly rotated secrets and revoked unused keys.
- Enabled GitHub Dependabot for automated security fixes.
- Used
Final Checklist for CI/CD Security
Category | Action |
---|---|
Access Management | Minimized GITHUB_TOKEN permissions |
Enforced branch protection | |
Secrets Handling | Used GitHub Secrets instead of plaintext |
Enabled encryption and secret scanning | |
Workflow Security | Pinned action versions |
Restricted permissions for workflows | |
Environment Protection | Required approvals for deployments |
Implemented wait timers to avoid rapid rollouts | |
Runner Security | Used GitHub-hosted runners |
Ensured fresh environments per job |
The manner we dealt with rollbacks and logging was critical too. Setting up build failure alerts and maintaining proper documentation helped our team stay on track.
OpenID Connect (OIDC) proved excellent for cloud access, better than storing long-term credentials. The security improvement was worth the extra setup time.
Regular audits of workflow permissions (quite conventional but necessary) helped us maintain security as our project grew. The requirements were specific, but the results were worth the effort.
🚀 Setting Up Your First GitHub Actions Workflow
GitHub Actions uses YAML-based workflows to automate tasks like CI/CD, testing, and deployments. While the syntax may seem complex initially, breaking it down into smaller building blocks makes it manageable.
Setting up our first workflow wasn’t straightforward (YAML syntax can be tricky). The building blocks seemed complex at first, but breaking them down made everything clearer.
Where to Place Workflow Files
You can use either .yml
or .yaml
extensions (both work the same way). The structure turned out quite conventional:
├── .github/ │ ├── workflows/ │ │ ├── deploy.yml │ │ ├── test-and-build.yaml
All workflow files must be stored inside the .github/workflows/
directory.
Basic Workflow Structure
A GitHub Actions workflow has four key sections:
Section | Purpose |
---|---|
Name | A descriptive name for the workflow (appears in the GitHub Actions tab). |
Events | Defines what triggers the workflow (e.g., push, pull request, cron). |
Jobs | Groups of tasks that execute on separate machines. |
Steps | The individual tasks that a job performs. |
Here’s a simple workflow that worked for us:
name: PixelPipeline # 1️⃣ Descriptive name on: # 2️⃣ Events (Triggers) push: branches: - main pull_request: branches: - main jobs: # 3️⃣ Jobs (Tasks to Run) build: runs-on: ubuntu-latest steps: # 4️⃣ Steps (Commands to Execute) - name: Checkout Code uses: actions/checkout@v4 - name: Set up Node.js uses: actions/setup-node@v4 with: node-version: '1 - name: Install Dependencies run: npm install - name: Run Tests run: npm test
Breakdown of Each Section
The initial line is what defines the name of the flow.
>Events: What Triggers the Workflow?
The on:
field defines when the workflow runs.
- Trigger on every push to
main
:
on: push: branches: - main
Trigger on pull requests targeting main
:
on: pull_request: branches: - main
You could also schedule it to run everyday:
on: schedule: - cron: "0 0 * * *"
Also you could schedule it to run on dispatch:
on: workflow_dispatch:
Coming to the next part of the YAML:
Jobs: Running Tasks in Parallel
The jobs:
section defines independent units of work. Each job runs on a separate virtual machine (runner).
The requirements were specific – each workflow needed events, jobs, and steps. The manner they dealt with marketplace actions saved us from writing everything from scratch.
jobs: build: runs-on: ubuntu-latest
Steps: Commands to Run in a Job
Steps define what happens inside a job.
Each step can:
✅ Use an action (prebuilt GitHub utility)
✅ Run a shell command (run:
)
✅ Store artifacts (files between jobs)
steps: - name: Checkout Code uses: actions/checkout@v4 # Fetches the repository - name: Set up Node.js uses: actions/setup-node@v4 with: node-version: '18' - name: Install Dependencies run: npm install - name: Run Tests run: npm test
Organizing Workflows: Best Practices
✔️ 1. Use Multiple Jobs for Parallel Execution
Instead of one giant job, split tasks into separate jobs for faster execution.
Example:
jobs: lint: runs-on: ubuntu-latest steps: - uses: actions/checkout@v4 - run: npm run lint test: runs-on: ubuntu-latest steps: - uses: actions/checkout@v4 - run: npm test
This approach is recommend as it :
✅ Runs faster (parallel jobs)
✅ Isolates failures (if linting fails, tests can still run)
Utilizing caches whenever possible – this improves performance:
- name: Cache Node.js modules uses: actions/cache@v4 with: path: ~/.npm key: ${{ runner.os }}-node-${{ hashFiles('**/package-lock.json') }} restore-keys: | ${{ runner.os }}-node-
Picking Workflow Triggers
Choosing when workflows run was critical for our setup. The common triggers that worked for us:
- Push events: Runs when code changes hit specific branches
- Pull request actions: Triggers during PR lifecycle
- Scheduled runs: Uses cron for timing
- Manual starts: Runs through GitHub interface
The configuration options proved flexible. For example, running only on Python file changes:
on: push: paths: - '**.py' # Python files only (saved us processing time)
First-time contributors need maintainer approval for running workflows. The security measures were quite conventional but necessary for managing our automated processes.
The trigger combinations offered plenty of options. Cost was another factor though – we had to be specific about which events triggered workflows to keep resource usage efficient.
Building Our CI Pipeline
Testing and quality checks turned out to be critical for our CI pipeline. The requirements were quite conventional – we needed reliable code verification that would catch issues early.
Setting Up Tests
Testing formed the foundation of our CI setup. GitHub Actions worked well with our various testing frameworks (quite conventional at the time). The process kicks off automatically whenever developers push code changes.
Our testing approach included:
- Test runners matching our programming language
- Unit tests checking individual parts
- Integration tests (these took some time to get right)
- End-to-end workflow validation
The isolated test environments proved excellent for consistent results across platforms. Our developers got quick feedback about their code changes, which helped catch issues early.
Build Automation
The build process (another critical piece) runs whenever code changes hit our repository. We structured our builds around several key elements:
Build Setup: Everything happens automatically – compiling code, getting dependencies, creating artifacts. The standardization really cut down our manual work and mistakes.
Build Machines: GitHub Actions gave us options for different operating systems – Linux, macOS, Windows, even ARM and containers. The consistency across platforms was exactly what we needed.
Multiple Builds: Running tests across different systems simultaneously saved us considerable time (being a startup, this mattered a lot).
Quality Standards
Quality checks went beyond basic testing, helping maintain our code standards:
Coverage Checks: These measurements showed which code parts needed more testing. The insights helped us focus our testing efforts where needed.
Security Tests: CodeQL scans our main branch after merges. The security verification (even with all its complexity) proved worth the setup time.
Code Style: Automated linters keep our code consistent. The manner they caught issues early saved us many review headaches.
Live Updates: The real-time logs made troubleshooting much simpler. Our team could spot and fix issues quickly.
GitHub keeps this check data for 400 days, giving us good visibility into our testing history. The status checks on pull requests show pending, passing, or failing states right next to commits (quite helpful during reviews).
The early error detection really improved our development speed. Cost was another factor – catching bugs early saved us considerable debugging time later.
Setting Up Continuous Deployment
Getting deployments right was the toughest part of our CI/CD journey. GitHub Actions helped us build a solid pipeline moving code from development to production, though the setup took considerable trial and error.
Deployment Environments
Setting up deployment targets proved tricky at first. We needed separate spaces for development, staging, and production. GitHub environments offered exactly what we needed:
Protection Rules:
- Deployment approvals (saved us from many potential issues)
- Time delays between deployments
- Strict branch and tag controls
Environment Setup:
- Secret storage for sensitive data
- Configuration variables management
- URL tracking for live systems
Deployment Control: The manner GitHub Actions handles concurrent deployments was excellent. It maintains:
- Single active deployment
- One deployment waiting
- Automatic cleanup of old deployment attempts
🚀 Implementing a Blue-Green Deployment Strategy Using GitHub Actions
Continuous Deployment (CD) requires a structured release strategy to ensure zero downtime and quick rollbacks in case of failures. One of the best ways to achieve this is through Blue-Green Deployment, which ensures a smooth transition between different versions of your application.
🔹 What is Blue-Green Deployment?
Blue-Green Deployment is a deployment strategy that maintains two separate environments:
Environment | Purpose |
---|---|
Blue | Live environment (currently serving users). |
Green | Staging environment (receives new updates before switching live). |
At any point:
✅ The Blue environment serves real traffic.
✅ The Green environment is tested with new updates.
✅ If the Green environment passes testing, traffic is switched from Blue → Green.
✅ If an issue arises, we quickly roll back to the previous environment.
We had the action flow setup as:
name: Blue-Green Deployment on: push: branches: - main # Deploys when pushing to the main branch jobs: deploy-green: name: Deploy to Green Environment runs-on: ubuntu-latest steps: - name: Checkout code uses: actions/checkout@v4 - name: Set up environment variables run: echo "DEPLOY_ENV=green" >> $GITHUB_ENV - name: Deploy Application run: ./deploy.sh green # Runs your deployment script - name: Run Tests run: ./run-tests.sh # Ensures the new version works correctly - name: Notify on Success if: success() run: echo "Green environment is ready!" switch-live: name: Switch Traffic to Green needs: deploy-green # Runs only if 'deploy-green' succeeds runs-on: ubuntu-latest steps: - name: Update Load Balancer (Switch Blue → Green) run: ./switch-traffic.sh green - name: Notify Deployment Completion run: echo "Traffic switched to Green. Deployment successful!" rollback: name: Rollback if Failure needs: deploy-green runs-on: ubuntu-latest if: failure() # Executes if 'deploy-green' fails steps: - name: Revert Traffic Back to Blue run: ./switch-traffic.sh blue - name: Notify Rollback run: echo "Rollback to Blue environment completed!"
Branch Management:
- Feature branches for changes
- Continuous testing for stability
- Security and performance monitoring
- Protection rules integration
Deployment Security:
- OpenID Connect for cloud access
- Branch protection for production
- Required reviews for changes
Recovery Process: The rollback capabilities saved us several times:
- Quick problem detection
- Version rollback options
- Backup restore process
Deployments start automatically when our code meets the requirements. Each release creates its own deployment record, helping us track everything properly.
The 30-day deployment delay option gave us time for thorough testing. Being a startup, we appreciated having admin overrides for urgent situations.
The automation cut our manual deployment work significantly. Cost was another factor – reducing human errors through automation proved worth the initial setup time. The speed improvement was remarkable, without compromising our quality standards.
Final Thoughts
GitHub Actions proved excellent for our deployment needs. The automation cut our release time significantly, while keeping our code quality high (something we struggled with before).
The requirements were quite conventional at the time – we needed reliable workflows, good testing, and secure deployments. GitHub’s marketplace saved us from writing everything from scratch (a relief for our small team). The logging and rollback features helped us recover quickly when things went wrong.
Setting up the pipeline wasn’t complex once we understood the basics. Our team (even those new to DevOps) got comfortable with it quickly. The combination of automated tests and quality checks gave us confidence in our deployments.
The manner they handled various aspects of CI/CD was really amazing. Cost was another factor – being a startup, we had to be working under a limited budget, but the features we got were worth the investment. Starting small and expanding gradually worked well for us, and we’d recommend the same approach for your team.
FAQs
Q1. What are the key components of a GitHub CI/CD pipeline? A GitHub CI/CD pipeline typically consists of workflow configuration files, jobs and steps that define specific tasks, event triggers that initiate the pipeline, and runners that execute the workflows. These components work together to automate the building, testing, and deployment of code.
Q2. How do I set up my first GitHub Actions workflow? To set up your first GitHub Actions workflow, create a YAML file in the .github/workflows
directory of your repository. Define the workflow name, specify triggers (like push or pull request events), and outline jobs with their respective steps. Use proper YAML syntax and indentation to structure your workflow file correctly.
Q3. What security best practices should I follow when setting up a GitHub CI/CD pipeline? Implement access control management, use GitHub Secrets for sensitive data, pin actions to specific versions, set up environment protection rules, and use GitHub-hosted runners for public repositories. Regularly audit workflow permissions and implement OpenID Connect for cloud resource access to enhance security.
Q4. How can I implement automated testing in my CI pipeline? Set up automated testing by configuring test runners for your programming language, implementing unit tests, integration tests, and end-to-end tests. Use GitHub Actions to automatically execute these tests whenever code changes are pushed to the repository, providing immediate feedback on potential issues.
Q5. What are some effective release strategies for continuous deployment? Effective release strategies include blue-green deployments, which maintain two identical production environments for easy rollbacks, and release branch management for grouping related changes. Implement deployment protection rules, use automated rollbacks for quick recovery from issues, and leverage GitHub environments for managing different deployment stages.